Create a game like Flappy Bird in Android using AndEngine

Keywords: AndEngine AndEnginePhysicsBox2DExtension SimpleBaseGameActivity ResourceManager SceneManager MenuScene CameraScene AutoParallaxBackground AnimatedSprite DynamicSpriteBatch TiledSprite Sound Music HUD Font PhysicsHandler GenericPool PhysicsWorld Fixture Body ContactListener
Contents- Overview
- Create a new Eclipse Android project
- Add AndEngine library
- Add AndEngine Physics Box2D extension
- Android manifest
- AndEngine concepts
- Game Activity
- Resource Manager
- TextureAtlas & TextureRegion
- Font
- Sound & Music
- Scene Manager
- Base Scene
- Splash Scene
- Main Menu Scene
- Sub Menu Scene
- Game Scene
- Auto Parallax Background
- HUD
- Bird
- Pipes
- Generic Pool
- Physics World
- Body & Fixture
- Contact Listener
- Camera Scene
- What's next?
17. Game Scene
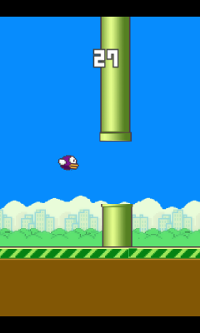
Let's first take a look at the basic structure of the new class for game scene. The class additionally implements IOnSceneTouchListener interface.
public class GameScene extends BaseScene implements IOnSceneTouchListener { @Override public void createScene() { mEngine.registerUpdateHandler(new FPSLogger()); setOnSceneTouchListener(this); //TODO create entities //TODO create PhysicsWorld //TODO create body and fixture /* The actual collision-checking. */ registerUpdateHandler(new IUpdateHandler() { @Override public void reset() {} @Override public void onUpdate(float pSecondsElapsed) { //TODO implement } }); //TODO create CameraScene for 'get ready' //TODO create CameraScene for 'game over' //TODO create HUD for score } @Override public boolean onSceneTouchEvent(Scene pScene, TouchEvent pSceneTouchEvent) { //TODO implement return false; } @Override public void onBackKeyPressed() { mSceneManager.setScene(SceneType.SCENE_MENU); } @Override public SceneType getSceneType() { return SceneType.SCENE_GAME; } @Override public void disposeScene() { //TODO } }We register an update handler that gets called on each update of the scene. So it is a good place to put logic that need to be executed repeatedly on each update like checking collisions, etc.
We'll discuss each item in detail in subsequent sections.
18. Auto Parallax Background
The auto-moving background is created using AutoParallaxBackground. We've already used it before in main menu scene. However, in game scene we will actually make the background move by setting non-zero value to pParallaxChangePerSecond.The sky and ground are parallax entity attached to the background. It's possible to give different velocity to each parallax entity using pParallaxFactor. Let's see how it's done in code.
private AutoParallaxBackground mAutoParallaxBackground; @Override public void createScene() { //... mAutoParallaxBackground = new AutoParallaxBackground(0, 0, 0, 10); mAutoParallaxBackground.attachParallaxEntity(new ParallaxEntity(-5.0f, new Sprite(0, SCREEN_HEIGHT - mResourceManager.mParallaxLayerBack.getHeight(), mResourceManager.mParallaxLayerBack, mVertexBufferObjectManager))); mAutoParallaxBackground.attachParallaxEntity(new ParallaxEntity(-10.0f, new Sprite(0, SCREEN_HEIGHT - mResourceManager.mParallaxLayerFront.getHeight(), mResourceManager.mParallaxLayerFront, mVertexBufferObjectManager))); setBackground(mAutoParallaxBackground); //... }To make the sky and ground move at different speed relative to the bird to create a parallax effect.
19. HUD
HUD stands for heads-up display. It stays at the same position on the screen and usually used for displaying score or game controls. HUD is a subclass of the Scene class.Here are the changes to the GameScene for displaying score.
private Text mHudText; private int score; private int most; @Override public void createScene() { //... most = mActivity.getMaxScore(); //create HUD for score HUD gameHUD = new HUD(); // CREATE SCORE TEXT mHudText = new Text(SCREEN_WIDTH/2, 50, mResourceManager.mFont5, "0123456789", new TextOptions(HorizontalAlign.LEFT), mVertexBufferObjectManager); mHudText.setText("0"); mHudText.setX((SCREEN_WIDTH - mHudText.getWidth()) / 2); mHudText.setVisible(false); gameHUD.attachChild(mHudText); mCamera.setHUD(gameHUD); }
Notice the Text is initialized with all the digits which speeds up rendering during runtime when actual score is set.
We have to make few changes in GameActivity as well to store the maximum score. Add this piece of code in GameActivity class.public int getMaxScore() { return getPreferences(Context.MODE_PRIVATE).getInt("maxScore", 0); } public void setMaxScore(int maxScore) { getPreferences(Context.MODE_PRIVATE).edit().putInt("maxScore", maxScore).commit(); }Later we will add code to update current score and max score while implementing the game play.
20. Bird

private AnimatedSprite mBird; @Override public void createScene() { //... //create entities final float birdX = (SCREEN_WIDTH - mResourceManager.mBirdTextureRegion.getWidth()) / 2 - 50; final float birdY = (SCREEN_HEIGHT - mResourceManager.mBirdTextureRegion.getHeight()) / 2 - 30; mBird = new AnimatedSprite(birdX, birdY, mResourceManager.mBirdTextureRegion, mVertexBufferObjectManager); mBird.setZIndex(10); mBird.animate(200); attachChild(mBird); //... }We've set Z-index to a high value so that the bird appears on top of other entities.