Create an elegantly designed Reminder/Alarm clock application

Keywords: ListActivity SimpleCursorAdapter SQLiteDatabase AlarmManager NotificationManager IntentService BroadcastReceiver ToggleButton ViewSwitcher DatePicker TimePicker RadioGroup
Contents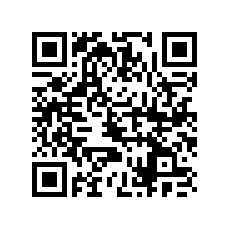

- Overview
- Create a new Eclipse Android project
- Define the Data model
- The Android Manifest file
- The Application class
- The Preferences screen
- The Alarm Service
- The Alarm Receiver
- The Alarm Setter
- The Main screen
- The Options Menu
- The Context Menu
- The Edit Dialog
- The New Reminder screen
- Date and Time Controls
8. The Alarm Receiver
The AlarmReceiver class is a simple BroadcastReceiver in which we override the onReceive() method to create a notification.public class AlarmReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { long id = intent.getLongExtra("id", 0); String msg = intent.getStringExtra("msg"); Notification n = new Notification(R.drawable.ic_launcher, msg, System.currentTimeMillis()); PendingIntent pi = PendingIntent.getActivity(context, 0, new Intent(), 0); n.setLatestEventInfo(context, "Remind Me", msg, pi); // TODO check user preferences n.defaults |= Notification.DEFAULT_VIBRATE; n.sound = Uri.parse(RemindMe.getRingtone()); // n.defaults |= Notification.DEFAULT_SOUND; n.flags |= Notification.FLAG_AUTO_CANCEL; NotificationManager nm = (NotificationManager) context.getSystemService(Context.NOTIFICATION_SERVICE); nm.notify((int)id, n); } }We need to declare the receiver in AndroidManifest.xml file just like any other Android component.
9. The Alarm Setter
We need another BroadcastReceiver for recreating the alarms when the device is rebooted. This is due to the fact that the Android system loses existing alarms on device reboot.public class AlarmSetter extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { Intent service = new Intent(context, AlarmService.class); service.setAction(AlarmService.CREATE); context.startService(service); } }The thing that makes it work is in the AndroidManifest.xml file. Notice that the receiver was declared to listen to the android.intent.action.BOOT_COMPLETED action. Additionally, it requires the android.permission.RECEIVE_BOOT_COMPLETED permission.
10. The Main screen
Now we are ready to develop the user interface of the application.The main screen can be broadly divided into three sections vertically. The menu bar, the date range selector, and the list view. Here is the main.xml layout file.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" android:background="@drawable/bg_repeat" > <RelativeLayout android:id="@+id/menu_bar" android:layout_width="fill_parent" android:layout_height="wrap_content" android:background="@color/dark_green" > <TextView android:id="@+id/heading_tv" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_alignParentTop="true" android:text="Remind Me" /> <ImageButton android:id="@+id/imageButton1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentRight="true" android:layout_alignParentTop="true" android:onClick="onClick" android:background="@null" android:src="@drawable/preferences" /> <ImageButton android:id="@+id/imageButton2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentTop="true" android:layout_toLeftOf="@id/imageButton1" android:onClick="onClick" android:background="@null" android:src="@drawable/add" /> </RelativeLayout> <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:background="@drawable/range" android:gravity="center_vertical" > <ImageButton android:id="@+id/imageButton3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:onClick="onClick" android:background="@null" android:src="@drawable/left" /> <TextView android:id="@+id/range_tv" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <ImageButton android:id="@+id/imageButton4" android:layout_width="wrap_content" android:layout_height="wrap_content" android:onClick="onClick" android:background="@null" android:src="@drawable/right" /> </LinearLayout> <LinearLayout android:id="@+id/list_view" android:layout_width="fill_parent" android:layout_height="0dip" android:layout_weight="1" android:orientation="vertical" > <ListView android:id="@android:id/list" android:layout_width="fill_parent" android:layout_height="fill_parent" android:divider="@drawable/line" android:dividerHeight="3dip" android:listSelector="@android:color/transparent" android:cacheColorHint="#00000000" android:drawSelectorOnTop="false" /> <TextView android:id="@android:id/empty" android:layout_width="fill_parent" android:layout_height="fill_parent" android:gravity="center" android:text="No data" /> </LinearLayout> </LinearLayout>Take some time to go through the content. We will skip few UI related stuff like tiling backgrounds, shape drawables, text effects, etc. which we have covered in an earlier article.
Next we'll create the activity which uses this layout file.