Build your first pro Android application with Eclipse

Keywords: AppCompat Fragment ActionBar ViewPager Navigation Drawer LoaderManager SQLiteDatabase CursorLoader SimpleCursorAdapter ContentProvider SQLiteOpenHelper ContentResolver ListFragment ListView GridView DialogFragment Volley Library RequestQueue ImageLoader NetworkImageView
Contents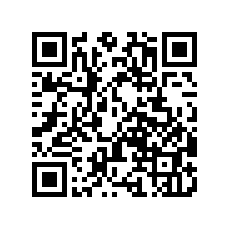

- Overview
- Create a new Eclipse Android project
- Add Android Support library
- Android manifest
- Application theme
- Application class
- Splash screen
- Login screen
- Help screen
- Settings screen
- Navigation Drawer
- Main screen
- Dialog Fragment
- Content Provider
- Data model
- List Fragment
- Data Adapter
- Grid View
- View Pager
- Detail screen
- Volley Library
- What's next?
18. Grid View
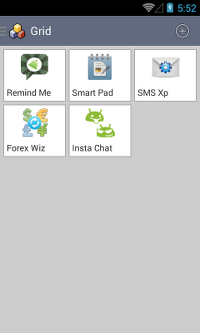
Create a new ItemGridFragment class as follows.
public class ItemGridFragment extends Fragment implements AdapterView.OnItemClickListener, LoaderManager.LoaderCallbacks<Cursor> { private OnFragmentInteractionListener mListener; private DataAdapter adapter; public ItemGridFragment() { // Required empty public constructor } @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout for this fragment View rootView = inflater.inflate(R.layout.fragment_grid, container, false); GridView gridview = (GridView) rootView.findViewById(R.id.gridview); adapter = new DataAdapter(getActivity(), R.layout.fragment_item_grid); gridview.setAdapter(adapter); gridview.setOnItemClickListener(this); getLoaderManager().initLoader(0, null, this); return rootView; } @Override public void onAttach(Activity activity) { super.onAttach(activity); try { mListener = (OnFragmentInteractionListener) activity; } catch (ClassCastException e) { throw new ClassCastException(activity.toString() + " must implement OnFragmentInteractionListener"); } } @Override public void onDetach() { super.onDetach(); mListener = null; } @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { if (null != mListener) { // Notify the active callbacks interface (the activity, if the // fragment is attached to one) that an item has been selected. mListener.onItemSelected(id); } } @Override public Loader<Cursor> onCreateLoader(int id, Bundle args) { CursorLoader loader = new CursorLoader(getActivity(), DataProvider.CONTENT_URI_DATA, new String[]{DataProvider.COL_ID, DataProvider.COL_CONTENT}, null, null, null); return loader; } @Override public void onLoadFinished(Loader<Cursor> loader, Cursor data) { adapter.swapCursor(data); } @Override public void onLoaderReset(Loader<Cursor> loader) { adapter.swapCursor(null); } }The use of LoaderManager.LoaderCallbacks, OnFragmentInteractionListener, and DataAdapter is exactly same as discussed earlier. We additionally implement AdapterView.OnItemClickListener interface for handling item click events.
<?xml version="1.0" encoding="utf-8"?> <GridView xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/gridview" android:layout_width="fill_parent" android:layout_height="fill_parent" android:padding="5dp" android:numColumns="3" android:verticalSpacing="5dp" android:horizontalSpacing="5dp" android:gravity="center" />
You can learn more about Grid View from Android Developers site.
Also, we have to create a new layout fragment_item_grid.xml for a grid item. It's similar to fragment_item_list.xml that we discussed earlier for list view.<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:padding="5dp" android:background="@drawable/background_item"> <com.android.volley.toolbox.NetworkImageView android:id="@+id/imageView1" android:layout_width="48dp" android:layout_height="48dp" android:layout_gravity="center_horizontal" /> <TextView android:id="@android:id/text1" android:layout_width="match_parent" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceSmall" android:gravity="center_vertical" android:paddingTop="6dip" android:singleLine="true" /> </LinearLayout>We have reused the DataAdapter class and OnFragmentInteractionListener interface created previously. However, let's revisit the code just for reference.
public class DataAdapter extends SimpleCursorAdapter { public DataAdapter(Context context, int layout) { super(context, layout, null, new String[]{DataProvider.COL_CONTENT}, new int[]{android.R.id.text1}, 0); } @Override public void bindView(View view, Context context, Cursor cursor) { String content = cursor.getString(cursor.getColumnIndex(DataProvider.COL_CONTENT)); Data data = new Data(content); String title = data.getTitle(); String iconUrl = data.getIcon(); TextView titleText = (TextView) view.findViewById(android.R.id.text1); titleText.setText(title); NetworkImageView iconView = (NetworkImageView) view.findViewById(R.id.imageView1); iconView.setDefaultImageResId(R.drawable.ic_launcher); iconView.setImageUrl(iconUrl, App.getInstance().getImageLoader()); } }
public interface OnFragmentInteractionListener { /** * Callback for when an item has been selected. */ public void onItemSelected(long id); }Next, we'll see how to use ViewPager view provided by Android support library.