Create a game like Flappy Bird in Android using AndEngine

Keywords: AndEngine AndEnginePhysicsBox2DExtension SimpleBaseGameActivity ResourceManager SceneManager MenuScene CameraScene AutoParallaxBackground AnimatedSprite DynamicSpriteBatch TiledSprite Sound Music HUD Font PhysicsHandler GenericPool PhysicsWorld Fixture Body ContactListener
Contents- Overview
- Create a new Eclipse Android project
- Add AndEngine library
- Add AndEngine Physics Box2D extension
- Android manifest
- AndEngine concepts
- Game Activity
- Resource Manager
- TextureAtlas & TextureRegion
- Font
- Sound & Music
- Scene Manager
- Base Scene
- Splash Scene
- Main Menu Scene
- Sub Menu Scene
- Game Scene
- Auto Parallax Background
- HUD
- Bird
- Pipes
- Generic Pool
- Physics World
- Body & Fixture
- Contact Listener
- Camera Scene
- What's next?
12. Scene Manager
The SceneManager class is designed to be a singleton so that it can manage all scenes in the game. Here is a basic outline of the class.public class SceneManager { private static final SceneManager INSTANCE = new SceneManager(); public enum SceneType {SCENE_SPLASH, SCENE_MENU, SCENE_GAME} private BaseScene mSplashScene; private BaseScene mMenuScene; private BaseScene mGameScene; private SceneType mCurrentSceneType; private BaseScene mCurrentScene; private SceneManager() {} public static SceneManager getInstance() { return INSTANCE; } public void setScene(SceneType sceneType) { switch (sceneType) { case SCENE_MENU: setScene(createMenuScene()); break; case SCENE_GAME: setScene(createGameScene()); break; case SCENE_SPLASH: setScene(createSplashScene()); break; } } private void setScene(BaseScene scene) { ResourceManager.getInstance().mActivity.getEngine().setScene(scene); mCurrentScene = scene; mCurrentSceneType = scene.getSceneType(); } public SceneType getCurrentSceneType() { return mCurrentSceneType; } public BaseScene getCurrentScene() { return mCurrentScene; } public BaseScene createSplashScene() { //TODO implement return mSplashScene; } private BaseScene createMenuScene() { //TODO implement return mMenuScene; } private BaseScene createGameScene() { //TODO implement return mGameScene; } }There is a create method for each scene. The setScene() method calls a specific create method corresponding to a scene.
We have to implement the empty create methods to return an instance of Scene. Ideally, the scene instances should be created once and reset before reuse. However, for simplicity we choose to recreate the instance every time a scene is set.
13. Base Scene
BaseScene will be our abstract super class for all scenes. First, create a package com.appsrox.flappychick.scene for holding all scenes in the game.Create a class inheriting from Scene and define few member variables that are commonly used.
public abstract class BaseScene extends Scene { protected final int SCREEN_WIDTH = GameActivity.CAMERA_WIDTH; protected final int SCREEN_HEIGHT = GameActivity.CAMERA_HEIGHT; protected GameActivity mActivity; protected Engine mEngine; protected Camera mCamera; protected VertexBufferObjectManager mVertexBufferObjectManager; protected ResourceManager mResourceManager; protected SceneManager mSceneManager; public BaseScene() { mResourceManager = ResourceManager.getInstance(); mActivity = mResourceManager.mActivity; mVertexBufferObjectManager = mActivity.getVertexBufferObjectManager(); mEngine = mActivity.getEngine(); mCamera = mEngine.getCamera(); mSceneManager = SceneManager.getInstance(); createScene(); } public abstract void createScene(); public abstract void onBackKeyPressed(); public abstract SceneType getSceneType(); public abstract void disposeScene(); }The abstract methods must be implemented by the sub types. Most of these methods would be invoked in SceneManager but onBackKeyPressed() needs to be tied to GameActivity for back button behaviour in a particular scene.
Add this code in GameActivity class.
@Override public void onBackPressed() { if (mSceneManager.getCurrentScene() != null) { mSceneManager.getCurrentScene().onBackKeyPressed(); return; } super.onBackPressed(); }
14. Splash Scene
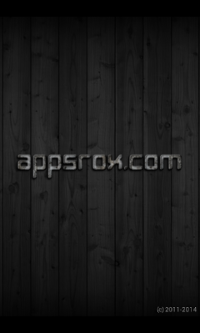
Create a class inheriting from BaseScene and implement the abstract methods.
public class SplashScene extends BaseScene { @Override public void createScene() { Sprite splash = new Sprite(0, 0, mResourceManager.mSplashTextureRegion, mVertexBufferObjectManager) { @Override protected void preDraw(GLState pGLState, Camera pCamera) { super.preDraw(pGLState, pCamera); pGLState.enableDither(); } }; attachChild(splash); Text copyrightText = new Text(0, 0, mResourceManager.mFont1, "(c) 2011-2014", new TextOptions(HorizontalAlign.LEFT), mVertexBufferObjectManager); copyrightText.setPosition(SCREEN_WIDTH - copyrightText.getWidth()-5, SCREEN_HEIGHT - copyrightText.getHeight()-5); attachChild(copyrightText); } @Override public void onBackKeyPressed() { mActivity.finish(); } @Override public SceneType getSceneType() { return SceneType.SCENE_SPLASH; } @Override public void disposeScene() { } }The important piece of code is in createScene() method where we create a sprite and text, and attach as child to the scene. Notice the use of Font resource in creating the Text object.
Sprite and Text are sub types of Entity. We need to specify position of an entity in a scene. Since we are using GLES2 branch hence origin is at the left-top of screen.
If you choose to use GLES2-AnchorCenter branch then remember that origin is in the lower left.